React Datta PRO#
React Datta Able PRO is the premium version of the admin dashboard template by CodedThemes, offering enhanced features and exclusive components beyond the standard version. This comprehensive React-based solution is designed for building sophisticated admin interfaces with minimal development effort.
👉 React Datta Able PRO - Product page
👉 React Datta Able PRO - Live Demo
👉 New to App-Generator? Sign IN with GitHub or Generate Web Apps in no time (free service).
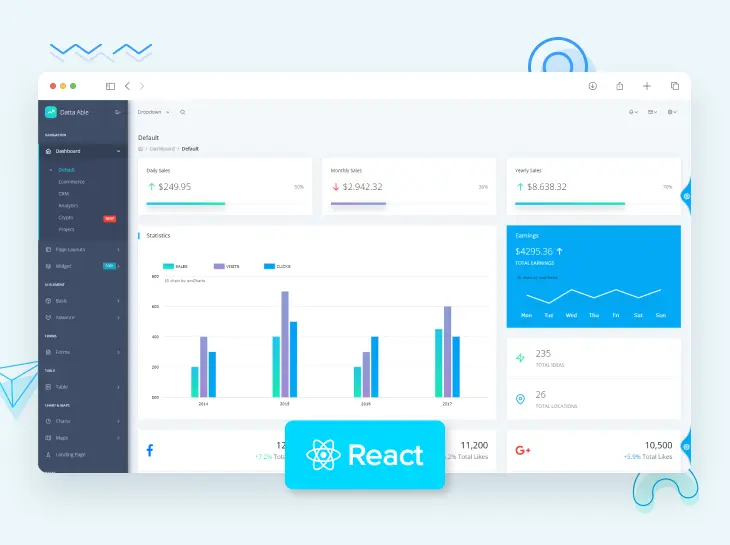
Enhanced PRO Features#
The PRO version expands on the base template with several premium additions:
Advanced authentication flows including social login
10+ pre-built dashboard variations (compared to 5+ in the basic version)
1500+ page templates (compared to 800+ in the basic version)
Enterprise-ready components like advanced data tables and charts
Premium support and regular updates
Ready-to-use applications including mail, chat, and kanban boards
Ready-to-Use Applications#
The PRO version includes complete applications that you can integrate directly:
// Example of using the Mail app component
import React from 'react';
import { MailApp } from '../pro-components/Apps';
const MailDashboard = () => {
return (
<MailApp
mailboxes={['inbox', 'sent', 'drafts', 'spam']}
onCompose={handleCompose}
onMailSelect={handleMailSelect}
// Additional configuration options
/>
);
};
Advanced Authentication#
The PRO version provides comprehensive authentication solutions:
// Example of social authentication integration
import React from 'react';
import { SocialAuth } from '../pro-components/Auth';
const LoginPage = () => {
return (
<div className="auth-wrapper">
<div className="auth-content">
{/* Regular login form */}
<form>
{/* Form fields */}
</form>
{/* Social authentication */}
<SocialAuth
providers={['google', 'facebook', 'twitter']}
onSocialLogin={handleSocialLogin}
/>
</div>
</div>
);
};
Project Structure#
The PRO version has an optimized project structure for large-scale applications:
src/
├── assets/ # Static assets
├── components/ # Basic components
│ └── pro/ # PRO-only components
├── config/ # Configuration files
├── layouts/ # Layout components
├── redux/ # State management
├── routes/ # Route definitions
├── services/ # API services
├── utils/ # Utility functions
├── views/ # Page components
│ └── apps/ # Full application views
└── App.js # Main application
Datta Able PRO provides a comprehensive solution for building complex admin interfaces, offering significant time savings and professional features that would otherwise require extensive custom development.
Links#
👉 New to App-Generator? Join our 10k+ Community using GitHub One-Click SignIN.
👉
Download
products and start fast a new project👉 Bootstrap your startUp, MVP or Legacy project with a custom development sprint