CoreUI#
CoreUI is a robust, enterprise-grade admin template built on top of Bootstrap. It distinguishes itself through its modular architecture, comprehensive component library, and strong focus on real-world application needs.
π New to App-Generator? Sign IN with GitHub or Generate Web Apps in no time (free service).
The template is designed for building responsive, cross-browser web applications while maintaining high performance and accessibility standards.
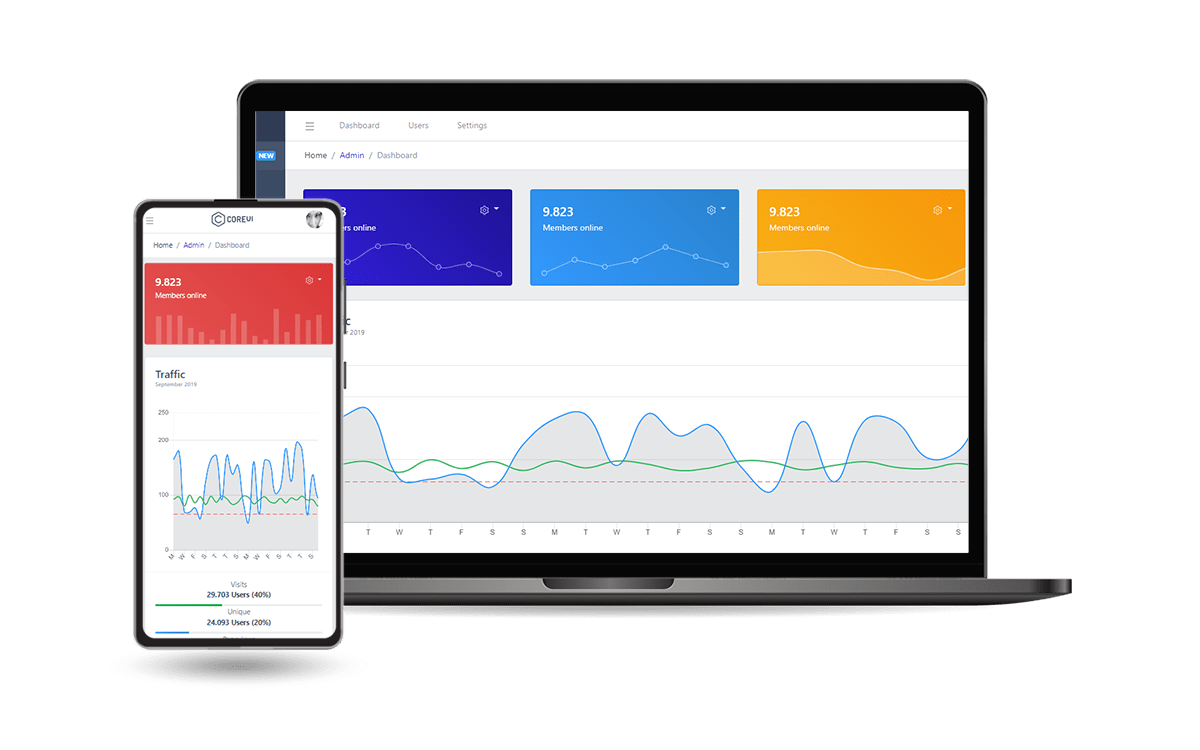
Project Structure#
CoreUI follows a well-organized directory structure:
coreui/
βββ dist/ # Compiled production files
βββ src/
β βββ assets/
β β βββ brand/ # Logo and brand assets
β β βββ icons/ # CoreUI Icons
β β βββ img/ # Images
β βββ js/
β β βββ core/ # Core functionality
β β βββ widgets/ # Widget components
β β βββ main.js # Main JavaScript file
β βββ pug/ # Pug templates (optional)
β βββ scss/
β β βββ style.scss # Main style file
β β βββ _custom.scss
β β βββ _variables.scss
β β βββ vendors/ # Third-party styles
β βββ views/ # HTML templates
βββ build/ # Build scripts
βββ node_modules/ # Dependencies
Core Layout Structure#
Hereβs the fundamental layout structure for CoreUI pages:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>CoreUI</title>
<!-- CoreUI CSS -->
<link href="css/style.css" rel="stylesheet">
</head>
<body class="c-app">
<!-- Sidebar -->
<div class="c-sidebar c-sidebar-dark c-sidebar-fixed c-sidebar-lg-show" id="sidebar">
<div class="c-sidebar-brand">
<img class="c-sidebar-brand-full" src="assets/brand/coreui-base.svg" height="46">
<img class="c-sidebar-brand-minimized" src="assets/brand/coreui-signet.svg" height="46">
</div>
<ul class="c-sidebar-nav">
<!-- Sidebar navigation items -->
</ul>
<button class="c-sidebar-minimizer c-class-toggler" type="button"></button>
</div>
<!-- Main content wrapper -->
<div class="c-wrapper">
<!-- Header -->
<header class="c-header c-header-light c-header-fixed">
<button class="c-header-toggler" type="button">
<span class="c-header-toggler-icon"></span>
</button>
<ul class="c-header-nav ml-auto">
<!-- Header navigation items -->
</ul>
</header>
<!-- Main content -->
<div class="c-body">
<main class="c-main">
<div class="container-fluid">
<!-- Page content -->
</div>
</main>
</div>
<!-- Footer -->
<footer class="c-footer">
<div>CoreUI Β© 2024</div>
<div class="ml-auto">Powered by CoreUI</div>
</footer>
</div>
</body>
</html>
Component System#
Card Components#
CoreUI extends Bootstrapβs card component with additional features:
<div class="card">
<div class="card-header">
Featured
<div class="card-header-actions">
<a class="card-header-action btn-setting" href="#">
<i class="cil-settings"></i>
</a>
<a class="card-header-action btn-minimize" href="#">
<i class="cil-arrow-circle-top"></i>
</a>
<a class="card-header-action btn-close" href="#">
<i class="cil-x"></i>
</a>
</div>
</div>
<div class="card-body">
Content
</div>
</div>
Customization#
SCSS Variables#
CoreUI provides extensive customization through SCSS variables:
// Core variables
$sidebar-width: 256px;
$sidebar-minimized-width: 56px;
$sidebar-brand-height: 56px;
// Theme colors
$primary: #321fdb;
$secondary: #ced2d8;
$success: #2eb85c;
$info: #39f;
$warning: #f9b115;
$danger: #e55353;
// Layout options
$enable-sidebar-nav-rounded: true;
$enable-footer-fixed: true;
$enable-header-fixed: true;
$enable-sidebar-fixed: true;
// Typography
$font-family-base: -apple-system, BlinkMacSystemFont, "Segoe UI", Roboto, "Helvetica Neue";
$font-size-base: 0.875rem;
JavaScript Configuration#
CoreUIβs behavior can be customized through JavaScript:
// Initialize CoreUI
window.addEventListener('load', function() {
coreui.Sidebar._jQueryInterface.call($('#sidebar'), 'show');
// Custom sidebar configuration
var sidebar = new coreui.Sidebar('#sidebar', {
minimize: true,
unfoldable: false,
breakpoints: {
xs: 'c-sidebar-show',
sm: 'c-sidebar-sm-show',
md: 'c-sidebar-md-show',
lg: 'c-sidebar-lg-show',
xl: 'c-sidebar-xl-show'
}
});
});
// Configure tooltip options globally coreui.Utils.setTooltipDistance(10);
Advanced Features#
State Management#
CoreUI implements a robust state management system:
// State management for sidebar
class SidebarState {
constructor() {
this.minimized = false;
this.unfoldable = false;
this.mobile = window.innerWidth < 992;
}
toggle() {
this.minimized = !this.minimized;
document.body.classList.toggle('c-sidebar-minimized');
}
// Persist state
save() {
localStorage.setItem('sidebar-state', JSON.stringify({
minimized: this.minimized,
unfoldable: this.unfoldable
}));
}
}
const sidebarState = new SidebarState();
Custom Events#
CoreUI provides custom events for component interactions:
// Listen for sidebar events
const sidebar = document.querySelector('#sidebar');
sidebar.addEventListener('classtoggle.coreui.sidebar', function(event) {
console.log('Sidebar class toggled:', event.detail);
});
sidebar.addEventListener('minimize.coreui.sidebar', function(event) {
console.log('Sidebar minimized:', event.detail);
});
Performance Optimization#
Lazy Loading#
Implement lazy loading for better performance:
// Lazy load components
document.addEventListener('DOMContentLoaded', function() {
const lazyComponents = document.querySelectorAll('[data-load]');
const loadComponent = (element) => {
const componentUrl = element.dataset.load;
fetch(componentUrl)
.then(response => response.text())
.then(html => {
element.innerHTML = html;
element.removeAttribute('data-load');
});
};
const observer = new IntersectionObserver((entries) => {
entries.forEach(entry => {
if (entry.isIntersecting) {
loadComponent(entry.target);
observer.unobserve(entry.target);
}
});
});
lazyComponents.forEach(component => observer.observe(component));
});
Asset Optimization#
// Configure webpack for optimal asset loading
module.exports = {
optimization: {
splitChunks: {
chunks: 'all',
cacheGroups: {
vendors: {
test: /[\\/]node_modules[\\/]/,
priority: -10
},
default: {
minChunks: 2,
priority: -20,
reuseExistingChunk: true
}
}
}
}
};
## Responsive Design
CoreUI implements a mobile-first approach:
// Responsive breakpoints
$grid-breakpoints: (
xs: 0,
sm: 576px,
md: 768px,
lg: 992px,
xl: 1200px,
xxl: 1400px
);
// Responsive utilities
@include media-breakpoint-down(md) {
.c-sidebar {
position: fixed;
z-index: $zindex-fixed + 1;
transform: translateX(-100%);
&.c-sidebar-show {
transform: translateX(0);
}
}
}
Best Practices#
Component Organization: - Keep components modular and reusable - Follow CoreUIβs naming conventions - Maintain consistent component structure - Document component dependencies
Performance Guidelines: - Minimize HTTP requests - Optimize asset loading - Use proper caching strategies - Implement lazy loading where appropriate
Accessibility: - Maintain ARIA labels - Ensure keyboard navigation - Provide sufficient color contrast - Support screen readers
Browser Support#
CoreUI supports all modern browsers: - Chrome (latest) - Firefox (latest) - Safari (latest) - Edge (latest) - Opera (latest) - Internet Explorer 11 (with polyfills)
Development Workflow#
# Install dependencies
npm install
# Start development server
npm run serve
# Build for production
npm run build
# Run tests
npm test
Resources and Support#
Official Documentation: [CoreUI Documentation](https://coreui.io/docs)
GitHub Repository: [CoreUI GitHub](coreui/coreui)
Support Forums: [CoreUI Community](https://community.coreui.io/)
Updates: Regular releases through npm
Remember to keep your CoreUI installation updated for the latest features and security patches.
Links#
π New to App-Generator? Join our 10k+ Community using GitHub One-Click SignIN.
π Download products and start fast a new project
π Bootstrap your startUp, MVP or Legacy project with a custom development sprint