Datta Able#
Open-Source Flask Template built with a minimum set of features on top of Datta Able, a modern dashboard design from CodedThemes. This template can be used to start a new project quickly by adding new features on top of the existing ones or simply for learning purposes.
👉 Datta Able Flask - Product Page (contains download link)
👉 Datta Able Flask - LIVE Demo
👉 Get Support via Email and Discord
👉 New to App-Generator? Join our 10k+ Community using GitHub One-Click SignIN.
Features#
Simple, Easy-to-Extend codebase
Datta Able Full Integration
Bootstrap 5 Styling
Session-based Authentication
DB Persistence: SQLite (default), can be used with MySql, PgSql
Docker
CI/CD integration for Render
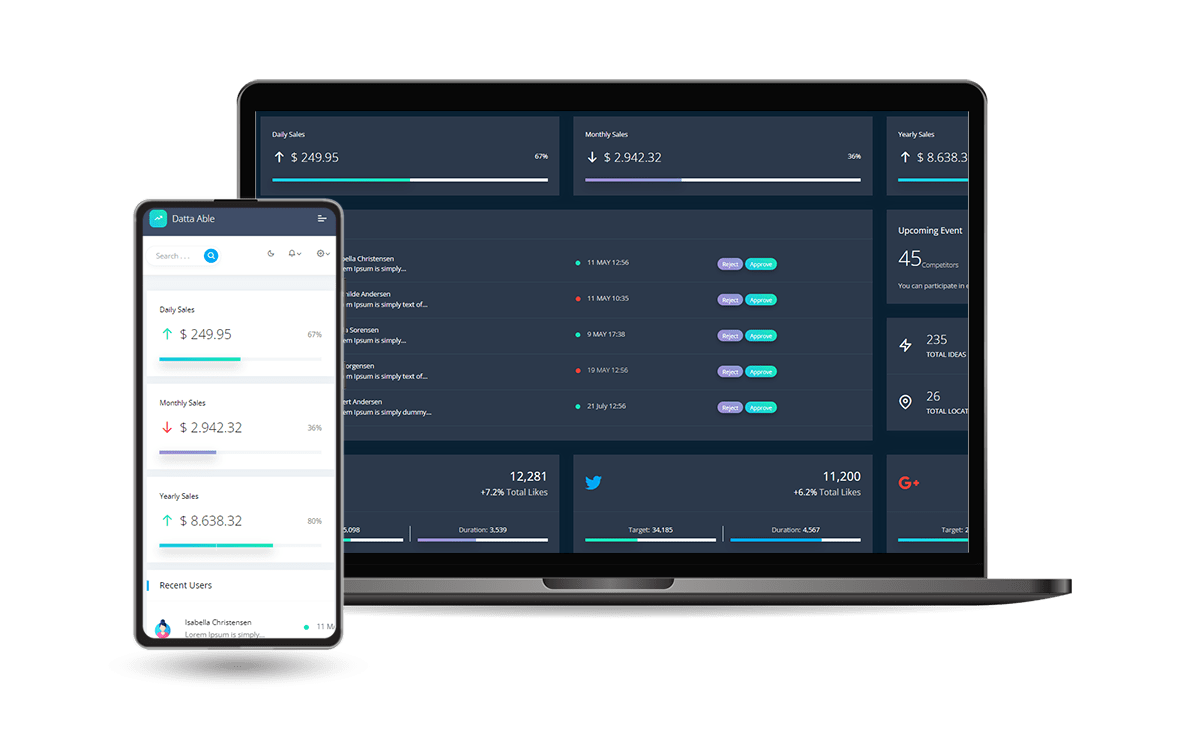
Prerequisites#
A few tools need to be installed in the system to use the starter efficiently:
Download Source Code#
The product can be downloaded from the official product page or directly from GitHub (public repository)
git clone https://github.com/app-generator/flask-datta-able.git
cd flask-datta-able
Once the source code is unzipped, the next step is to start it and use provided features.
Start in Docker#
The fastest way to start the product is to execute the Docker set up:
docker-compose up --build
If Docker is properly installed in the system, you can visit the browser at http://localhost:5085. Product should be up and running.
Codebase#
The project is coded using a simple and intuitive structure presented below:
Core: holds the project settings
Home: the application that integrates the Datta Able Design
Api: the generated API
< PROJECT ROOT >
|
|-- apps/
| |
| |-- home/ # A simple app that serve HTML files
| | |-- routes.py # Define app routes
| |
| |-- authentication/ # Handles auth routes (login and register)
| | |-- routes.py # Define authentication routes
| | |-- models.py # Defines models
| | |-- forms.py # Define auth forms (login and register)
| |
| |-- static/
| | |-- <css, JS, images> # CSS files, Javascripts files
| |
| |-- templates/ # Templates used to render pages
| | |-- includes/ # HTML chunks and components
| | | |-- navigation.html # Top menu component
| | | |-- sidebar.html # Sidebar component
| | | |-- footer.html # App Footer
| | | |-- scripts.html # Scripts common to all pages
| | |
| | |-- layouts/ # Master pages
| | | |-- base-fullscreen.html # Used by Authentication pages
| | | |-- base.html # Used by common pages
| | |
| | |-- accounts/ # Authentication pages
| | | |-- login.html # Login page
| | | |-- register.html # Register page
| | |
| | |-- home/ # UI Kit Pages
| | |-- index.html # Index page
| | |-- 404-page.html # 404 page
| | |-- .html # All other pages
| |
| config.py # Set up the app
| __init__.py # Initialize the app
|
|-- requirements.txt # App Dependencies
|
|-- .env # Inject Configuration via Environment
|-- run.py # Start the app - WSGI gateway
Manual Build#
It’s best to use a Python Virtual Environment for installing the project dependencies. You can use the following code to create the virtual environment
virtualenv env
To activate the environment execute env\Scripts\activate.bat for Windows or source env/bin/activate on Linux-based operating systems.
Having the VENV active, we can proceed and install the project dependencies:
pip install -r requirements.txt
Environment#
The starter loads the environment variables from .env file. Here are the critical ones:
DEBUG: set by default to False (development mode)
SECRET_KEY: a random value used by Django to secure sensitive information like passwords and cookie information
- Database Credentials: DB_ENGINE, DB_USERNAME, DB_PASS, DB_HOST, DB_PORT, DB_NAME
if detected, the database is switched automatically from the default SQLite to the specified DBMS
Setting up the Database#
By default, the application uses SQLite for persistence. In order to use MySql / PostgreSQL, you’ll need to install the Python driver(s):
pip install mysqlclient # for MySql
# OR
pip install psycopg2 # for PostgreSQL
To connect the application with the database, you’ll need to fill in the credentials int the .env file and run the migrations.
DB_ENGINE=mysql
# OR
DB_ENGINE=postgresql
# DB credentials below
DB_HOST=localhost
DB_NAME=<DB_NAME_HERE>
DB_USERNAME=<DB_USER_HERE>
DB_PASS=<DB_PASS_HERE>
DB_PORT=3306
Set up the environment
export FLASK_APP=run.py
Use the following commands to set up the database:
# Init migration folder
flask db init # to be executed only once
flask db migrate # Generate migration SQL
flask db upgrade # Apply changes
Running the project#
The following command starts the project using Flask development server:
flask run # Starts on default PORT 5000
flask run --port 8999 # Starts on PORT 8999 (custom port)
By default Flask starts on port 5000 but this can be easily changed by adding the PORT number as argument. At this point, the app runs at http://127.0.0.1:5000/
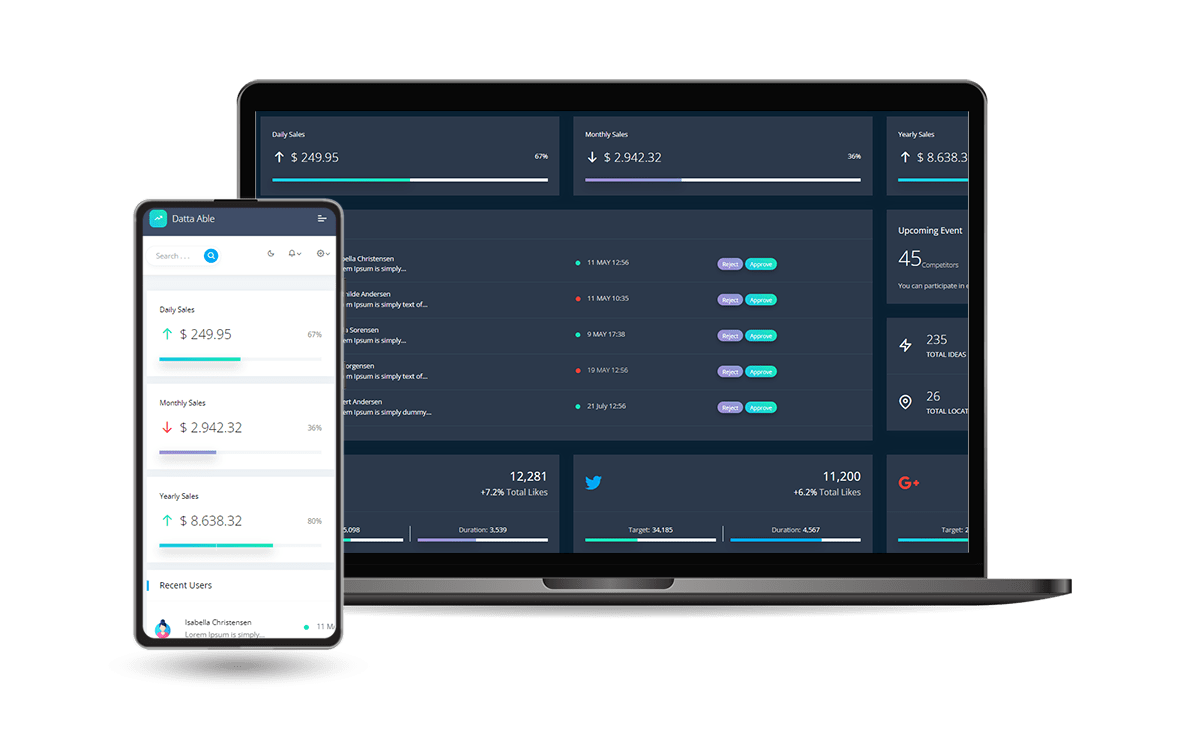
Create Users#
By default, the app redirects guest users to authenticate. In order to access the private pages, follow this set up:
Start the app
Access the registration page and create a new user: - http://127.0.0.1:5000/register/
Access the sign in page and authenticate - http://127.0.0.1:5000/login/